- Published on
Table in SwiftUI
- Authors
- Name
A container that presents rows of data arranged in one or more columns, optionally providing the ability to select one or more members.
一个提供多行数据,其中每行包括一列或者多列的容器。可选地提供选择一个或者多个成员的能力。
Overview
You commonly create tables from collections of data.The following example shows how to create a simple, three-column table from an array of Person instance that conform to the Identifiable protocol:
我们可以通过下面的代码来创建一个二维的表格
import SwiftUI
struct Person: Identifiable {
let givenName: String
let familyName: String
let emailAddress: String
let id = UUID()
var fullName: String {
givenName + " " + familyName
}
}
struct ContentView: View {
@State private var people = [
Person(givenName: "Juan", familyName: "Chavez", emailAddress: "[email protected]"),
Person(givenName: "Mei", familyName: "Chen", emailAddress: "[email protected]"),
Person(givenName: "Tom", familyName: "Clark", emailAddress: "[email protected]"),
Person(givenName: "Gita", familyName: "Kumar", emailAddress: "[email protected]")
]
var body: some View {
Table(people) {
TableColumn("Given Name", value: \.givenName)
TableColumn("Family Name", value: \.familyName)
TableColumn("E-Mail Address", value: \.emailAddress)
}
}
}
#Preview {
ContentView()
}
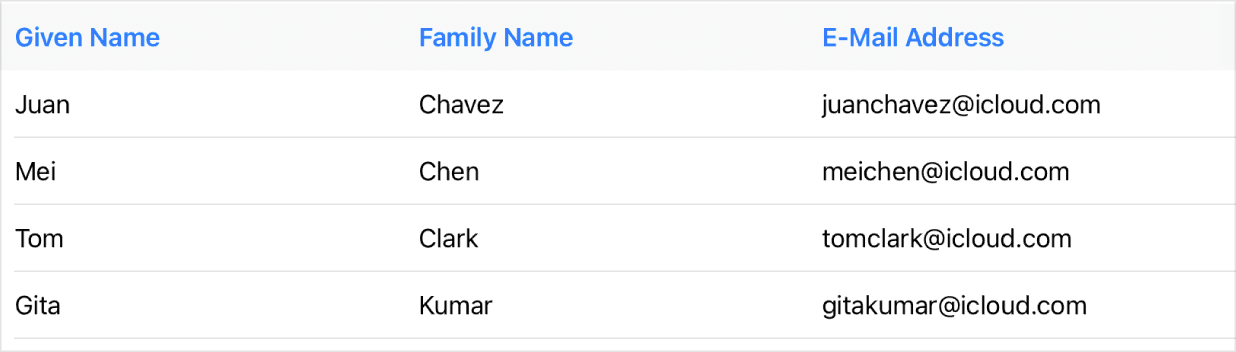
If there are more rows than can fit in the available space, Table provides vertical srolling automatically. On macOS,the table also provides horizontal scrolling if there are more columns than can fit in the width of the view. Scroll bars appear as needed on iOS; on macOS, the Table shows or hides scroll bars based on the "Show scroll bars" system preference.
如果超过了行数可显示的空间,Table会自动提供垂直滚动条。在 macOS 上,如果表格的列数超过视图宽度的显示范围,表格还支持水平滚动。在iOS上滚动条根据需要显示,在macOS上,滚动条根据"show scroll bar"系统偏好设置来显示或隐藏。
Supporting selection in tables
To make rows of a table selectable, provide a binding to a selection variable. Binding to a single instance of the table data's id type creates a single-selection table. Binding to a Set creates a table that supports multiple selections.
为了使表格的行可选,请为选择变量提供绑定。 将绑定设置为表格数据ID类型的单个实例,将创建一个单选表格。 将绑定设置为一个集合,将创建一个支持多选的表格。
The following example shows how to add multi-select to the previous example. A Text view below the table shows the number of items currently selected.
struct SelectableTable: View {
@State private var selectedPeople = Set<Person.ID>()
var body: some View {
Table(people, selection: $selectedPeople) {
TableColumn("Given Name", value: \.givenName)
TableColumn("Family Name", value: \.familyName)
TableColumn("E-Mail Address", value: \.emailAddress)
}
Text("\(selectedPeople.count) people selected")
}
}
如果只是选择单个,代码如下:
@State private var selectedPeople: UUID?
var body: some View {
Table(people,selection: $selectedPeople) {
TableColumn("Given Name", value: \.givenName)
TableColumn("Family Name", value: \.familyName)
TableColumn("E-Mail Address", value: \.emailAddress)
TableColumn("Full Name", value: \.fullName)
}
Text("\(String(describing: selectedPeople)) people selected")
}
其中需要关注的点就是如何传递selection这个参数,